The movie database is the API website where you can find a huge collection of movie data. You can also use all the movies database on your website or web page. For that, you only need to request the API key from OMDb API websites to get access to the all movies data.
Today I will show you the simplest way to use OMDb API in your website and how you can use the information to show your client. Here I will show you how you can code using Html and JavaScript to show attractive movie information. I have used bootstrap-3 for the responsive layout and I have also used somehow cascading Style to look better interface.
First of all, create an index.html file In this file, we will only code to create a search box with a button.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Movie</title>
<meta name="viewport" content="width=device-width, initial-scale=1">
<meta name="theme-color" content="#065af3">
<link rel="stylesheet" href="css/style.css?ver=1">
<script src="js/jquery-3.4.1.min.js"></script>
<link rel="stylesheet" href="css/bootstrap.min.css">
<script src="js/main.js?ver=4"></script>
<script src="js/bootstrap.min.js"></script>
</head>
<body>
<div class="container" id="home_search" style="margin-bottom: 50px;">
<div class="row">
<div class="col-lg-6 col-lg-offset-3">
<div id="need2hide">
<h1 class="text-center" style="color: white; margin-top: 180px; font-family: Lucida Calligraphy;">Search Movie Name</h1>
</div>
<div class="wrapper">
<form id="search_form">
<div class="input-data">
<input type="text" required="" id="search_text" autocomplete="off">
<label for="name">Search Movie</label>
</div>
<div class="text-center">
<button type="submit" class="search_btn">Search</button>
</div>
</form>
</div>
</div><!-- col lg end -->
</div>
</div>
<div class="container">
<div class="row" id="movie_list"></div>
</div>
</body>
</html>
Output

Now Let's code few codes in the style.css file for upper style on the main page.
a, a:link, a:visited,
a:hover, a:active{text-decoration: none;
color: currentColor;}
*{
margin: 0;
padding: 0;
outline: none;
box-sizing: border-box;
}
body{
min-height: 100vh;
background: linear-gradient(-135deg, #c850c0, #4158d0);
}
.wrapper{
background-color: #fff;
padding: 30px;
box-shadow: 0px 0px 10px rgba(0, 0, 0, 0.1);
}
.wrapper .input-data{
height: 40px;
width: 100%;
position: relative;
}
.wrapper .input-data input{
height: 100%;
width: 100%;
border: none;
font-size: 17px;
border-bottom: 2px solid silver;
transition: all 1s ease;
font-weight: bold;
text-align: center;
}
.wrapper .input-data input:focus{
border-bottom: 2px solid red;
}
.input-data input:focus ~ label,
.input-data input:valid ~ label{
transform: translateY(-20px);
font-size: 15px;
color: #4158d0;
}
.wrapper .input-data label{
position: absolute;
bottom: 10px;
left: 0;
color: grey;
pointer-events: none;
transition: all 0.3s ease;
}
.search_btn{
background-image: linear-gradient(54deg, #53b2fe, #065af3)}
.search_btn{
margin-top: 20px;
padding: 10px 45px;
color: white;
box-shadow: 0 1px 7px 0 rgba(0, 0, 0, 0.2);
border-radius: 34px;
font-size: 24px;
line-height: 24px;
font-weight: 700;
border: none;
}
.search_btn:hover{
background-image: linear-gradient(54deg, #065af3, #53b2fe);
color: #fff;
text-decoration: none;
}
#movie_list img{height: 300px;
width: 220px;
overflow: hidden;
min-height: 300px;
box-shadow: 0 0 8px 2px rgba(0,0,0,0.5);
}
@media(max-width: 768px){
#progress{width: 200px !important;
}
}
Now it's time to code in the main.js file for submitting the form and retrieve data from the movie database API.
$(document).ready(function (event) {
$("#search_form").submit(function(event){
$("#need2hide").fadeOut();
event.preventDefault();
var movie = $("#search_text").val();
var url = "https://www.omdbapi.com?apikey=ea49aac1";
$.ajax({
method: 'GET',
url: url+"&s="+movie,
success: function (data) {
console.log(data)
console.log(data.Search);
var ifmovie = (data.Response);
if(ifmovie == "False"){alert("No Movie Available!");
message = `<div class="container"><div class="well">
<h2 class="text-center">Sorry 😲 No Movie Found !</h2>
<h3>Try to Search with another keyword!</h3>
<h3>You have not typed movie name</h3>
</div></div>`;
$("#movie_list").html(message);
}
var arr = data.Search;
var string = "";
for(var m = 0; m<arr.length; m++) {
console.log(arr[m].Title);
string += `
<div class="col-lg-3 col-xs-10 col-lg-offset-0 col-xs-offset-1" style="margin-top: 20px;">
<div class="well text-center">
<img src="${arr[m].Poster}" alt="Sorry! Poster Could Not Found" onerror="this.onerror=null; this.src='assets/not_available.png'">
<div style="height: 16px; padding: 20px 0px; overflow:hidden;">
<p>${arr[m].Title}</p>
</div>
<div class="text-center"><a href="movie_details.html?movie_id=${arr[m].imdbID}" type="button" class="btn btn-info">View More!</a></div>
</div>
</div>
`;
}
$("#movie_list").html(string);
}
});//*ajax process end
});/*form submit end*/
});
The above code will show only ten movies at a time if you want you to show 10 more movies you need to paste the below codes at the end of Ajax's request.
//load more 10 movies
$.ajax({
method: 'GET',
url: url+"&s="+movie+"&page=2",
success: function (data) {
console.log(data);
console.log(data.Search);
var arr = data.Search;
var string2 = "";
for(var m = 0; m<arr.length; m++) {
console.log(arr[m].Title);
string2 += `
<div class="col-lg-3 col-xs-10 col-lg-offset-0 col-xs-offset-1" style="margin-top: 20px;">
<div class="well text-center">
<img src="${arr[m].Poster}" alt="Sorry! Poster Could Not Found" onerror="this.onerror=null; this.src='assets/not_available.png'">
<div style="height: 16px; padding: 20px 0px; overflow:hidden;">
<p>${arr[m].Title}</p>
</div>
<div class="text-center"><a href="movie_details.html?movie_id=${arr[m].imdbID}" type="button" class="btn btn-info">View More!</a></div>
</div>
</div>
`;
}
$("#movie_list").append(string2);
}
});/*ajax process end*/
Now, After submitting the form after input any movie name it will show the output like the below image.
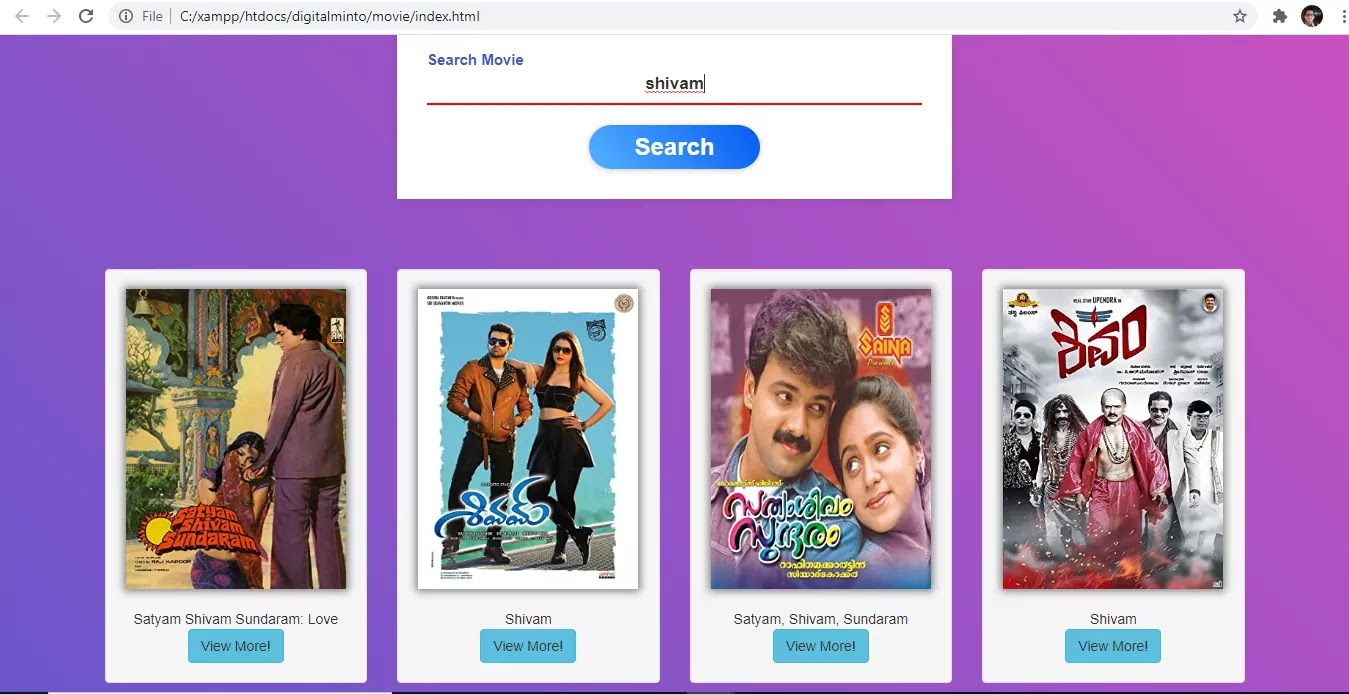
Finally, we have got all the movies list with the movie I'd we call also get more information from the movie I'd for that we need to create one more HTML page where we can show a specific movie's information which movie we have selected for that we need to create one more html page for example movie_detail.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Movie Details</title>
<meta name="viewport" content="width=device-width, initial-scale=1">
<meta name="theme-color" content="#065af3">
<link rel="stylesheet" href="css/style.css?ver=1">
<script src="js/jquery-3.4.1.min.js"></script>
<link rel="stylesheet" href="css/bootstrap.min.css">
<script src="js/main.js"></script>
<script src="js/bootstrap.min.js"></script>
<style>
body{color: white;}
</style>
</head>
<body>
<div class="container">
<div class="page-header" id="movie_title"></div>
<div class="row">
<div class="col-lg-3 col-lg-offset-0 col-xs-8 col-xs-offset-2">
<div id="poster"></div>
</div>
<div class="col-lg-9 col-lg-offset-0 col-xs-10 col-xs-offset-1">
<div id="movie"></div>
</div>
</div>
<div class="text-center" style="margin-bottom: 50px;">
<a href="index.html" class="search_btn" style="">Back To Search!</a>
</div>
</div>
<script>
$(document).ready(function (event) {
var urlParams = new URLSearchParams(window.location.search);
var movie_id = urlParams.get('movie_id');
/*alert(movie_id);*/
var url = "https://www.omdbapi.com?apikey=ea49aac1";
$.ajax({
method: 'GET',
url: url+"&i="+movie_id,
success: function (data) {
console.log(data);
var movie_details = `<h1 class="text-center">${data.Title}</h1>`;
$("#movie_title").html(movie_details);
var poster = `<div class="text-center"><img class="img-responsive" src="${data.Poster}" alt="No Movie Poster Available" onerror="this.onerror=null; this.src='assets/not_available.png'"></div>`;
$("#poster").html(poster);
var movie_rating = `
<div id="progress" class="container" style="width: 255px; margin-top: 20px;">
<div class="progress"><div class="progress-bar progress-bar-info progress-bar-striped active" role="progressbar" arial-valuenow="${data.imdbRating*10}" arial-valuemin="50" style="width: ${data.imdbRating*10}%;"><div style="color: #000;"><b>Ratings: ${data.imdbRating}</b></div></div></div>
</div>
`;
$("#poster").append(movie_rating);
var details = `
<h2>${data.Title}</h2>
<h3>${data.Genre}</h3>
<h3>Realse Date : ${data.Released}</h3>
<h3>Movie Runtime : ${data.Runtime}</h3>
<h3>Language : ${data.Language}</h3>
<h3>Production : ${data.Production}</h3>
<p style="font-size: 18px; margin-top: 20px;"><b>Actors</b> : ${data.Actors}</p>
<p style="font-size: 18px; margin-top: 20px;"><b style="font-size: 20px;">StoryLine</b><br>${data.Plot}<br>
<a href="https://www.imdb.com/title/${data.imdbID}/" target="_blank">Read More...</a></p>
<div class="text-center" style="margin-bottom: 50px;">
<p>Imdb Votes : ${data.imdbVotes}</p>
<a href="https://www.imdb.com/title/${data.imdbID}/" target="_blank" class="btn btn-warning">View in IMDB Website</a></div>
`
$("#movie").html(details);
}
});/*ajax process end*/
});
</script>
</body>
</html>
Final web page view for OMdb api.

If you still have problem in using OMDb movie Api comment below.